Sysadmins, these of us who run and handle Linux computer systems most carefully, have direct entry to instruments that assist us work extra effectively. To enable you to use these instruments to their most profit to make your life simpler, this collection of articles explores utilizing automation within the type of Bash shell scripts. It covers:
- The benefits of automation with Bash shell scripts
- Why utilizing shell scripts is a better option for sysadmins than compiled languages like C or C++
- Creating a set of necessities for brand spanking new scripts
- Creating easy Bash shell scripts from command-line interface (CLI) packages
- Enhancing safety via utilizing the consumer ID (UID) working the script
- Using logical comparability instruments to supply execution move management for command-line packages and scripts
- Using command-line choices to manage script performance
- Creating Bash capabilities that may be known as from a number of areas inside a script
- Why and methods to license your code as open supply
- Creating and implementing a easy take a look at plan
I beforehand wrote a collection of articles about Bash instructions and syntax and creating Bash packages on the command line, which you will discover within the references part on the finish of this text. But this collection of 4 articles is as a lot about creating scripts (and a few strategies that I discover helpful) as it’s about Bash instructions and syntax.
Why I take advantage of shell scripts
In Chapter 9 of The Linux Philosophy for Sysadmins, I write:
“A sysadmin is best when considering—interested by methods to resolve present issues and about methods to keep away from future issues; interested by methods to monitor Linux computer systems so as to discover clues that anticipate and foreshadow these future issues; interested by methods to make [their] job extra environment friendly; interested by methods to automate all of these duties that have to be carried out whether or not day-after-day or every year.
“Sysadmins are next most productive when creating the shell programs that automate the solutions that they have conceived while appearing to be unproductive. The more automation we have in place, the more time we have available to fix real problems when they occur and to contemplate how to automate even more than we already have.”
This first article explores why shell scripts are an vital instrument for the sysadmin and the fundamentals of making a quite simple Bash script.
Why automate?
Have you ever carried out a protracted and sophisticated activity on the command line and thought, “Glad that’s done. Now I never have to worry about it again!”? I’ve—often. I finally found out that nearly all the pieces that I ever must do on a pc (whether or not mine or one which belongs to an employer or a consulting buyer) will have to be executed once more someday sooner or later.
Of course, I at all times suppose that I’ll keep in mind how I did the duty. But, typically, the subsequent time is much sufficient into the long run that I overlook that I’ve ever executed it, not to mention how to do it. I began writing down the steps required for some duties on bits of paper, then thought, “How stupid of me!” So I transferred these scribbles to a easy notepad software on my laptop, till in the future, I assumed once more, “How stupid of me!” If I’m going to retailer this knowledge on my laptop, I would as properly create a shell script and retailer it in a normal location, like /usr/native/bin or ~/bin, so I can simply sort the title of the shell program and let it do all of the duties I used to do manually.
For me, automation additionally implies that I haven’t got to recollect or recreate the main points of how I carried out the duty so as to do it once more. It takes time to recollect methods to do issues and time to sort in all of the instructions. This can turn into a big time sink for duties that require typing giant numbers of lengthy instructions. Automating duties by creating shell scripts reduces the typing essential to carry out routine duties.
Shell scripts
Writing shell packages—also called scripts—is the most effective technique for leveraging my time. Once I write a shell program, I can rerun it as many instances as I must. I can even replace my shell scripts to compensate for adjustments from one launch of Linux to the subsequent, putting in new hardware and software program, altering what I need or want to perform with the script, including new capabilities, eradicating capabilities which are now not wanted, and fixing the not-so-rare bugs in my scripts. These sorts of adjustments are simply a part of the upkeep cycle for any sort of code.
Every activity carried out by way of the keyboard in a terminal session by coming into and executing shell instructions can and ought to be automated. Sysadmins ought to automate all the pieces we’re requested to do or determine must be executed. Many instances, doing the automation upfront saves me time the primary time.
One Bash script can comprise anyplace from a number of instructions to many 1000’s. I’ve written Bash scripts with just one or two instructions, and I’ve written a script with over 2,700 strains, greater than half of that are feedback.
Getting began
Here’s a trivial instance of a shell script and methods to create it. In my earlier collection on Bash command-line programming, I used the instance from each e book on programming I’ve ever learn: “Hello world.” From the command line, it appears like this:
[pupil@testvm1 ~]$ echo "Hello world"
Hello world
By definition, a program or shell script is a sequence of directions for the pc to execute. But typing them into the command line each time is kind of tedious, particularly when the packages are lengthy and sophisticated. Storing them in a file that may be executed with a single command saves time and reduces the likelihood for errors to creep in.
I like to recommend making an attempt the next examples as a non-root consumer on a take a look at system or digital machine (VM). Although the examples are innocent, errors do occur, and being protected is at all times sensible.
The first activity is to create a file to comprise your program. Use the contact command to create the empty file, good day, then make it executable:
[pupil@testvm1 ~]$ contact good day
[pupil@testvm1 ~]$ chmod 774 good day
Now, use your favourite editor so as to add the next line to the file:
echo "Hello world"
Save the file and run it from the command line. You can use a separate shell session to execute the scripts on this collection:
[pupil@testvm1 ~]$ ./good day
Hello world!
This is the best Bash program it’s possible you’ll ever create—a single assertion in a file. For this train, your full shell script can be constructed round this straightforward Bash assertion. The perform of this system is irrelevant for this function, and this straightforward assertion lets you construct a program construction—a template for different packages—with out caring concerning the logic of a purposeful function. You can focus on the essential program construction and creating your template in a quite simple approach, and you’ll create and take a look at the template itself slightly than a posh purposeful program.
Shebang
The single assertion works advantageous so long as you utilize Bash or a shell suitable with the instructions used within the script. If no shell is specified within the script, the default shell can be used to execute the script instructions.
The subsequent activity is to make sure that the script will run utilizing the Bash shell, even when one other shell is the default. This is completed with the shebang line. Shebang is the geeky strategy to describe the #! characters that explicitly specify which shell to make use of when working the script. In this case, that’s Bash, but it surely could possibly be some other shell. If the required shell is just not put in, the script is not going to run.
Add the shebang line as the primary line of the script, so now it appears like this:
#!/usr/bin/bash
echo "Hello world!"
Run the script once more—it is best to see no distinction within the outcome. If you could have different shells put in (comparable to ksh, csh, tcsh, zsh, and so forth.), begin one and run the script once more.
Scripts vs. compiled packages
When writing packages to automate—properly, all the pieces—sysadmins ought to at all times use shell scripts. Because shell scripts are saved in ASCII textual content format, they are often considered and modified by people simply as simply as they will by computer systems. You can look at a shell program and see precisely what it does and whether or not there are any apparent errors within the syntax or logic. This is a robust instance of what it means to be open.
I do know some builders take into account shell scripts one thing lower than “true” programming. This marginalization of shell scripts and people who write them appears to be predicated on the concept that the one “true” programming language is one which have to be compiled from supply code to supply executable code. I can let you know from expertise that that is categorically unfaithful.
I’ve used many languages, together with BASIC, C, C++, Pascal, Perl, Tcl/Expect, REXX (and a few of its variations, together with Object REXX), many shell languages (together with Korn, csh and Bash), and even some meeting language. Every laptop language ever devised has had one function: to permit people to inform computer systems what to do. When you write a program, whatever the language you select, you might be giving the pc directions to carry out particular duties in a selected sequence.
Scripts will be written and examined way more shortly than compiled languages. Programs normally have to be written shortly to satisfy time constraints imposed by circumstances or the pointy-haired boss. Most scripts that sysadmins write are to repair an issue, to scrub up the aftermath of an issue, or to ship a program that have to be operational lengthy earlier than a compiled program could possibly be written and examined.
Writing a program shortly requires shell programming as a result of it permits a fast response to the wants of the shopper—whether or not that’s you or another person. If there are issues with the logic or bugs within the code, they are often corrected and retested virtually instantly. If the unique set of necessities is flawed or incomplete, shell scripts will be altered in a short time to satisfy the brand new necessities. In common, the necessity for pace of growth within the sysadmin’s job overrides the necessity to make this system run as quick as doable or to make use of as little as doable in the way in which of system assets like RAM.
Most issues sysadmins do take longer to determine methods to do than to execute. Thus, it may appear counterproductive to create shell scripts for all the pieces you do. It takes a while to put in writing the scripts and make them into instruments that produce reproducible outcomes and can be utilized as many instances as needed. The time financial savings come each time you possibly can run the script with out having to determine (once more) methods to do the duty.
Final ideas
This article did not get very far with making a shell script, but it surely did create a really small one. It additionally explored the explanations for creating shell scripts and why they’re probably the most environment friendly possibility for the system administrator (slightly than compiled packages).
In the subsequent article, you’ll start making a Bash script template that can be utilized as a place to begin for different Bash scripts. The template will finally comprise a Help facility, a GNU licensing assertion, quite a few easy capabilities, and a few logic to take care of these choices, in addition to others that could be wanted for the scripts that can be primarily based on this template.
Resources
This collection of articles is partially primarily based on Volume 2, Chapter 10 of David Both’s three-part Linux self-study course, Using and Administering Linux—Zero to SysAdmin.
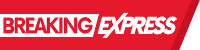