When you are programming, you are actually defining a process, or a routine, you need the pc to carry out. A easy analogy compares pc programming to baking bread: you checklist substances as soon as to arrange the work atmosphere, you then checklist the steps you should take to finish up with a loaf of bread. In each programming and baking, some steps should be repeated at completely different intervals. In baking bread, as an illustration, this might be the method of feeding a sourdough tradition:
STIR=100
SNOOZE=86400operate feed_culture
And later, kneading and proofing the dough:
KNEAD=600
SNOOZE=7200operate process_dough
remove_from(proofing_drawer)
knead($KNEAD)
return_to_drawer($SNOOZE)
In programming, these subroutines may be expressed as capabilities. Functions are essential for programmers as a result of they assist scale back redundancy in code, which in flip reduces the quantity of upkeep required. For instance, within the imaginary situation of baking bread programmatically, if you want to change the period of time the dough proofs, so long as you have used a operate earlier than, you merely have to alter the worth of the seconds as soon as, both by utilizing a variable (known as SNOOZE within the pattern code) or instantly within the subroutine that processes dough. That can prevent a number of time, as a result of you do not have to hunt via your codebase for each attainable point out of rising dough, a lot much less fear about lacking one. Many a bug’s been attributable to a missed worth that did not get modified or by a poorly executed sed
command in hopes of catching each final match with out having to hunt for them manually.
In Bash, defining a operate is as simple as setting it both within the script file you are writing or in a separate file. If you save capabilities to a devoted file, you’ll be able to supply
it into your script as you’ll embrace
a library in C or C++ or import
a module into Python. To create a Bash operate, use the key phrase operate
:
operate foo
Here’s a easy (and considerably contrived, as this might be made less complicated) instance of how a operate works with arguments:
#!/usr/bin/env bash
ARG=$1operate mimic
if [[ -z $ARG ]]; then
ARG='world'
fi
echo "hey $ARG"mimic $ARG
Here are the outcomes:
$ ./mimic
hey world
$ ./mimic all people
hey all people
Note the ultimate line of the script, which executes the operate. This is a typical level of confusion for starting scripters and programmers: capabilities do not get executed mechanically. They exist as potential routines till they’re known as.
Without a line calling the operate, the operate would solely be outlined and would by no means run.
If you are new to Bash, attempt executing the pattern script as soon as with the final line included and once more with the final line commented out.
Using capabilities
Functions are very important programming ideas, even for easy scripts. The extra comfy you turn into with capabilities, the higher off you will be once you’re confronted with a posh downside that wants one thing extra dynamic than simply declarative traces of instructions. Keeping general-purpose capabilities in separate information also can prevent some work, because it’ll assist you to construct up routines you generally use as a way to reuse them throughout initiatives. Look at your scripting habits and see the place capabilities would possibly match.
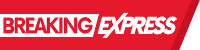