There are a few methods to be taught a programming language. If you are new to coding, you often be taught some fundamental laptop coding ideas and attempt to apply them. If you already know easy methods to code in one other language, you relearn how coding ideas are expressed within the new language.
In both case, a handy option to be taught these new ideas is to create a easy guessing recreation. This forces you to grasp how a language receives enter and sends output, the way it compares information, easy methods to management a program’s move, and easy methods to leverage conditionals to have an effect on an consequence. It additionally ensures that you understand how a language constructions its code; as an illustration, Lua or Bash can simply run as a script, whereas Java requires you to create a category.
In this text, I will display easy methods to implement a guessing recreation for the terminal in C++.
Install dependencies
To comply with together with this text, you want C++ and a compiler.
You can get every part you want on Linux by putting in the Qt Creator IDE out of your distribution’s software program repository.
On Fedora, CentOS, or RHEL:
$ sudo dnf set up qt-creator
On Debian, Ubuntu, Chromebook, or related:
$ sudo apt set up qtcreator
This article would not make the most of the Qt Creator IDE, however it’s a simple option to get every part you want put in, and for complicated C++ initiatives (together with these with a GUI), it is a vital software to have. On macOS or Windows, comply with the installation instructions on Qt’s web site.
Set up contains and namespace
C++’s core language is minimal. Even a easy software requires using extra libraries. This software makes use of iostream to achieve entry to the cout
and cin
key phrases.
Also, make sure that this system makes use of the std
namespace:
#embrace <iostream>utilizing namespace std;
This is not strictly mandatory, however with out setting the namespace to std
, all key phrases from the iostream library require a namespace prefix. For occasion, as an alternative of writing cout
, I must write std::cout
.
Statements in C++ terminate with a semicolon.
Create a operate
Every C++ software requires not less than one operate. The major operate of a C++ software should be referred to as foremost
, and it should return an integer (int
), which corresponds to the POSIX expectation that a course of returns zero upon success and one thing else upon failure. You create a brand new operate by offering its return kind after which its identify:
int foremost()
Implement program logic
The recreation code should first produce a random quantity for the participant to guess. You do that in C++ by establishing a seed for pseudo-random quantity technology. A easy seed is the present time. Once the seed begins, you retrieve a quantity between 1 and 100 by calling the rand
operate with an higher constraint of 100. This generates a random quantity from zero to 99, so add 1 to no matter quantity is chosen and assign the end result to a variable referred to as quantity
. You should additionally declare a variable to carry the participant’s guess. For readability, I am calling this variable guess
.
This pattern code additionally features a debug assertion that tells you precisely what the random quantity is. This is not excellent for a guessing recreation, however it makes testing rather a lot quicker. Later, you possibly can take away the road or simply remark it out by prefacing it with //
:
srand (time(NULL));
int quantity = rand() % 100+1;
int guess = zero;cout << quantity << endl; //debug
Add do-while and if statements
A do-while
assertion in C++ begins with the key phrase do
and encloses every part that you really want C++ to do in braces. Close the assertion with the whereas
key phrase adopted by the situation that should be met (in parentheses):
do whereas ( quantity != guess );
The recreation code happens inside an if
assertion with an else if
and else
statements to offer the participant with hints.
First, immediate the participant for a guess with a cout
assertion. The cout
operate prints output onto stdout
. Because the cout
assertion is not appended with the endl
(endline) operate, no linebreak happens. Immediately following this cout
assertion, inform C++ to attend for enter by utilizing the cin
operate. As you may surmise, cin
waits for enter from stdin
.
Next, this system enters the if
management assertion. If the participant’s guess is bigger than the pseudo-random quantity contained within the quantity
variable, then this system prints out a touch adopted by a newline character. This breaks the if
assertion, however C++ continues to be trapped throughout the do-while
loop as a result of its situation (the quantity
variable being equal to guess
) has not but been met.
If the participant’s guess is lower than the pseudo-random quantity contained within the quantity
variable, then this system prints out a touch adopted by a newline character. This once more breaks the if
assertion, however this system stays trapped throughout the do-while
loop.
When guess
is the same as quantity
, the important thing situation is lastly met, the else
assertion is triggered, the do-while
loop ends, and the applying ends:
do
cout << "Guess a number between 1 and 100: ";
cin >> guess;if ( guess > quantity) cout << "Too excessive.n" << endl;
else if ( guess < quantity )
else
cout << "That's proper!n" << endl;
exit(zero);
// fi
whereas ( quantity != guess );
return zero;
} // foremost
Building the code and taking part in the sport
You can construct your software with GCC:
$ g++ -o guess.bin guess.cpp
Run the binary to strive it out:
$ ./guess.bin
74
Guess a quantity between 1 and 100: 76
Too excessive.Guess a quantity between 1 and 100: 1
Too low.Guess a quantity between 1 and 100: 74
That's proper!
Success!
Give C++ a strive
The C++ language is complicated. Writing C++ purposes for terminals can train you a large number about information varieties, reminiscence administration, and code linking. Try writing a helpful utility in C++ and see what you possibly can uncover!
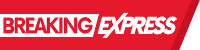