For a long time, builders have struggled with optimizing persistence layer implementation by way of storing enterprise knowledge, retrieving related knowledge rapidly, and—most significantly— simplifying knowledge transaction logic no matter programming languages.
Fortunately, this problem triggered the invention of Java ecosystems through which builders can implement the Java Persistence API (JPA). For occasion, Hibernate Object Relational Mapper (ORM) with Panache is the usual framework for JPA implementation within the Java ecosystem.
Kotlin is a programming language designed to run enterprise purposes with a number of programming languages on high of Java Virtual Machine (JVM) for the Java persistence implementation. But there’s nonetheless the hurdle for Java builders to make amends for the brand new syntax and JPA APIs of Kotlin.
This article will clarify how Quarkus makes it simpler for builders to implement Kotlin purposes via the Quarkus Hibernate ORM Panache Kotlin extension.
Create a brand new Hibernate ORM Kotlin mission utilizing Quarkus CLI
First, create a brand new Maven mission utilizing the Quarkus command-line device (CLI). The following command will add Quarkus extensions to allow Hibernate ORM Panache Kotlin
and PostgreSQL JDBC
extensions:
$ quarkus create app hibernate-kotlin-example
-x jdbc-postgresql, hibernate-orm-panache-kotlin
The output ought to appear to be this:
...
<[SUCCESS] ✅ quarkus mission has been efficiently generated in:
--> /Users/danieloh/Downloads/demo/hibernate-kotlin-example
...
Create a brand new entity and repository
Hibernate ORM with Panache permits builders to deal with entities with energetic file patterns with the next advantages:
- Auto-generation of IDs
- No want for getters/setters
- Super helpful static strategies for entry akin to
listAll()
anddiscover()
- No want for customized queries for fundamental operations (e.g.
Person.discover
(“name”, “daniel”))
Kotlin does not assist the Hibernate ORM with Panache in the identical method Java does. Instead, Quarkus permits builders to carry these capabilities into Kotlin utilizing the companion
object, as illustrated under:
@Entity(identify = "Person")
class Person : PanacheEntity() {
lateinit var identify: String
}
Here is a straightforward instance of how builders can implement PanacheRepository
to entry the entity:
@ApplicationScoped
class PersonRepository: PanacheRepository<Person> {
enjoyable findByName(identify: String) = discover("name", identify).firstResult()
}
Super easy! Now I’ll present you the best way to implement sources to entry knowledge by RESTful APIs.
Create a useful resource to deal with RESTful APIs
Quarkus totally helps context and dependency injection (CDI), which permits builders to inject PersonRepository
to entry the information (e.g., findByName(identify)
) within the sources. For instance:
@Inject
lateinit var personRepository: PersonRepository@GET
@Produces(MediaType.TEXT_PLAIN)
@Path("/{name}")
enjoyable greeting(@PathParam("name") identify: String): String {
return "Hello ${personRepository.findByName(name)?.name}"
}
Run and check the appliance
As all the time, run your Quarkus software utilizing Developer Mode:
$ cd hibernate-kotlin-example
$ quarkus dev
The output ought to appear to be this:
...
INFO [io.quarkus] (Quarkus Main Thread) Profile dev activated.
Live Coding activated.
INFO [io.quarkus] (Quarkus Main Thread)
Installed options: [agroal, cdi, hibernate-orm,
hibernate-orm-panache-kotlin, jdbc-postgresql,
kotlin, narayana-jta, resteasy, smallrye-context-propagation, vertx]--
Tests paused
Press [r] to renew testing, [o] Toggle check output,
[:] for the terminal, [h] for extra choices>
Quarkus Dev services arise a related database container mechanically if you run a container runtime (e.g., Docker or Podman) and add a database extension. In this instance, I already added the jdbc-postgresql
extension, so a PostgreSQL container will probably be operating mechanically when the Quarkus Dev mode begins. Find the answer in my GitHub repository.
Access the RESTful API (/hiya
) to retrieve the information by the identify
parameter. Execute the next curl
command line in your native terminal:
& curl localhost:8080/hiya/Daniel
The output ought to appear to be this:
Hello Daniel
Conclusion
This is a fundamental rationalization of how Quarkus permits builders to simplify JPA implementation utilizing Kotlin programming APIs for reactive Java purposes. Developers may also have higher developer experiences, akin to dev providers and dwell coding, whereas they preserve growing with Kotlin programming in Quarkus. For extra details about Quarkus, go to the Quarkus web page.
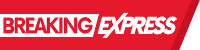