An utility written utilizing programming languages like C and C++ requires you to program the destruction of objects in reminiscence once they’re now not wanted. The extra your utility grows, the good the likelihood that you will overlook releasing unused objects. This results in a reminiscence leak and ultimately the system reminiscence will get used up, and sooner or later there is no additional reminiscence to allocate. This leads to a scenario the place the appliance fails with an OutOfMemoryError. But within the case of Java, Garbage Collection (GC) occurs robotically throughout utility execution, so it alleviates the duty of handbook deallocation and potential reminiscence leaks.
Garbage Collection is not a single job. The Java Virtual Machine (JVM) has eight completely different sorts of Garbage Collection, and it is helpful to know every one’s objective and energy.
1. Serial GC
A primitive implementation of GC utilizing only a single thread. When Garbage Collection occurs, it pauses the appliance (generally referred to as a “stop the world” occasion.) This is appropriate for functions that may stand up to small pauses. Garbage Collection has a small footprint, so that is the popular GC sort for embedded functions. This Garbage Collection model might be enabled at runtime:
$ java -XX:+UseSerialGC
2. Parallel GC
Like Serial GC, this additionally makes use of a “stop the world” technique. That implies that whereas GC is going on, utility threads are paused. But on this case, there are a number of threads performing GC operation. This sort of GC is appropriate for functions with medium to massive knowledge units operating in a multithreaded and multiprocessor surroundings.
This is the default GC in JVM, and is also referred to as the Throughput Collector. Various GC parameters, like throughput, pause time, variety of threads, and footprint, might be tuned with appropriate JVM flags:
- Number of threads:
-XX:ParallelGCThreads=<N>
- Pause time:
-XX:MaxGCPauseMillis=<N>
- Throughput (time spent for GC in comparison with precise utility execution):
-XX:GCTimeRatio=<N>
- Maximum heap footprint:
-Xmx<N>
- Parallel GC might be explicitly enabled:
java -XX:+UseParallelGC
. With this selection, minor GC within the younger technology is completed with a number of threads, however GC and compaction is completed with a single thread within the outdated technology.
There’s additionally a model of Parallel GC referred to as Parallel Old GC, which makes use of a number of threads for each younger and outdated generations:
$ java -XX:+UseParallelOldGC
3. Concurrent Mark Sweep (CMS)
Concurrent Mark Sweep (CMS) rubbish assortment is run alongside an utility. It makes use of a number of threads for each minor and main GC. Compaction for reside objects is not carried out in CMS GC after deleting the unused objects, so the time paused is lower than in different strategies. This GC runs concurrently with the appliance, which slows the response time of the appliance. This is appropriate for functions with low pause time. This GC was deprecated in Java 8u, and utterly faraway from 14u onwards. If you are still utilizing a Java model that has it, although, you may allow it with:
$ java -XX:+UseConcMarkSweepGC
In the case of CMS GC, the appliance is paused twice. It’s paused first when it marks a reside object that is immediately reachable. This pause is called the initial-mark. It’s paused a second time on the finish of the CMS GC part, to account for the objects that have been missed in the course of the concurrent cycle, when utility threads up to date the objects after CMS GC have been accomplished. This is called the comment part.
4. G1 (Garbage First) GC
Garbage first (G1) was meant to switch CMS. G1 GC is parallel, concurrent, and incrementally compacting, with low pause-time. G1 makes use of a distinct reminiscence format than CMS, dividing the heap reminiscence into equal sized areas. G1 triggers a world mark part with a number of threads. After the mark part is full, G1 is aware of which area could be largely empty and chooses that area for a sweep/deletion part first.
In the case of G1, an object that is greater than half a area measurement is taken into account a “humongous object.” These objects are positioned within the Old technology, in a area appropriately referred to as the humongous area. To allow G1:
$ java -XX:+UseG1GC
5. Epsilon GC
This GC was launched in 11u and is a no-op (do nothing) GC. Epsilon simply manages reminiscence allocation. It doesn’t do any precise reminiscence reclamation. Epsilon is meant solely when you realize the precise reminiscence footprint of your utility, and is aware of that it’s rubbish assortment free.
$ java -XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC
6. Shenandoah
Shenandoah was launched in JDK 12, and is a CPU intensive GC. It performs compaction, deletes unused objects, and launch free area to the OS instantly. All of this occurs in parallel with the appliance thread itself. To allow Shenandoah:
$ java -XX:+UnlockExperimentalVMOptions
-XX:+UseShenandoahGC
7. ZGC
ZGC is designed for functions which have low latency necessities and use massive heaps. ZGC permits a Java utility to proceed operating whereas it performs all rubbish assortment operations. ZGC was launched in JDK 11u and improved in JDK 12. Both Shenandoah and ZGC have been moved out of the experimental stage as of JDK 15. To allow ZGC:
$ java -XX:+UnlockExperimentalVMOptions -XX:+UseZGC
Flexible rubbish assortment
Java supplies flexibility for reminiscence administration. It’s helpful to get conversant in the completely different strategies obtainable so you may select what’s greatest for the appliance you are creating or operating.
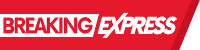