In the Java programming language, a hashmap is a listing of related values. Java makes use of hashmaps to retailer knowledge. If you retain a variety of structured knowledge, it is helpful to know the assorted retrieval strategies out there.
Create a hashmap
Create a hashmap in Java by importing and utilizing the HashMap
class. When you create a hashmap, you need to outline what constitutes a sound worth (corresponding to Integer
or String
). A hashmap holds pairs of values.
package deal com.opensource.instance;import java.util.HashMap;
public class Mapping {
public static void most important(String[] args) {
HashMap<String, String> myMap = new HashMap<>();
myMap.put("foo", "hello");
myMap.put("bar", "world");System.out.println(myMap.get("foo") + " " + myMap.get("bar"));
System.out.println(myMap.get("hello") + " " + myMap.get("world"));
}
}
The put
technique means that you can add knowledge to your hashmap, and the get
technique retrieves knowledge from the hashmap.
Run the code to see the output. Assuming you’ve got saved the code in a file known as most important.java
, you possibly can run it instantly with the java
command:
$ java ./most important.java
hiya world
null null
Calling the second values within the hashmap returns null
, so the primary worth is actually a key and the second a worth. This is named a dictionary or associative array in some languages.
You can combine and match the sorts of knowledge you set right into a hashmap so long as you inform Java what to anticipate when creating it:
package deal com.opensource.instance;import java.util.HashMap;
public class Mapping {
public static void most important(String[] args) {
HashMap<Integer, String> myMap = new HashMap<>();
myMap.put(71, "zombie");
myMap.put(2066, "apocalypse");System.out.println(myMap.get(71) + " " + myMap.get(2066));
}
}
Run the code:
$ java ./most important.java
zombie apocalypse
Iterate over a hashmap with forEach
There are some ways to retrieve all knowledge pairs in a hashmap, however probably the most direct technique is a forEach
loop:
package deal com.opensource.instance;import java.util.HashMap;
public class Mapping {
public static void most important(String[] args) {
HashMap<String, String> myMap = new HashMap<>();
myMap.put("foo", "hello");
myMap.put("bar", "world");// retrieval
myMap.forEach( (key, worth) ->
System.out.println(key + ": " + worth));
}
}
Run the code:
$ java ./most important.java
bar: world
foo: hiya
Structured knowledge
Sometimes it is sensible to make use of a Java hashmap so you do not have to maintain observe of dozens of particular person variables. Once you perceive easy methods to construction and retrieve knowledge in a language, you are empowered to generate complicated knowledge in an organized and handy means. A hashmap is not the one knowledge construction you will ever want in Java, however it’s a terrific one for associated knowledge.
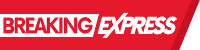