In the Java programming language, an array is an ordered assortment of knowledge. You can use an array to retailer data in a structured manner. It’s helpful to know the varied methods you may retrieve that information once you want it. It’s price noting that in Java, an associative array (additionally known as a dictionary in some languages) known as a hashmap. This article would not cowl hashmaps, however you may learn all about them in my Using a hashmap in Java article.
Creating a string array
The easiest array in Java is a one-dimensional array. It’s primarily an inventory. For an array of strings:
Notice that once you retrieve the info from an array, the index begins at 0, not 1. In different phrases, an array containing three values is numbered 0 to 2.
Alternatively, you may create an array and populate it later. When you do that, nonetheless, it’s essential to additionally inform Java the size of the array. The “length”, on this context, refers back to the variety of objects an array can maintain.
package deal com.opensource.instance;public class Example {
public static void primary(String[] args) {
String[] myArray = new String[3];System.out.println("Empty myArray created.");
// populate the array
myArray[0] = "foo";
myArray[1] = "bar";
myArray[2] = "baz";// retrieval
System.out.println(myArray[0]);
System.out.println(myArray[1]);
System.out.println(myArray[2]);
}
}
Assuming the code is saved in a file known as primary.java
, run it utilizing the java
command:
$ java ./primary.java
foo
bar
baz
To create an array of integers, outline the info kind as int
as a substitute of String
:
Run the code:
Iterating over an array
Once you’ve got saved information in an array, you most likely intend to retrieve it sooner or later. The most direct approach to see all information in an array is to create a for
loop that will get the size of the array utilizing the .size
methodology, after which loops over the array numerous instances equal to the size:
Run the code:
$ java ./primary.java
foo
bar
baz
Multidimensional arrays
An array would not must be only a easy checklist. It will also be an inventory of lists. This known as a multidimensional array, and it is fairly intuitive so long as you consider it as an array of arrays:
To see the contents of the array, you need to use a nested for
loop. In a one-dimensional array, you needed to acquire the size of myArray
so your for
loop knew when to cease iterating over the array. This time, it’s essential to acquire the size of every array inside myArray
. For simplicity, I name these two arrays outer
and internal
, with the previous being myArray
, and the internal
representing every nested array:
int outer = myArray.size;
int internal = myArray[1].size;
Once you could have each lengths, you employ them as the boundaries of your for
loop:
for (int i = 0; i < outer; i++) {
for (int j = 0; j < internal; j++) {
System.out.println(myArray[i][j]);
}
}
Here’s the total code pattern:
Run the code:
$ java ./primary.java
zombie
apocalypse
comfortable
halloween
Arrays of knowledge
Sometimes it makes extra sense to make use of a Java array than to trace dozens of particular person variables. Once you perceive methods to construction and retrieve information in a language, you may generate complicated information in an organized and handy manner.
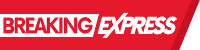